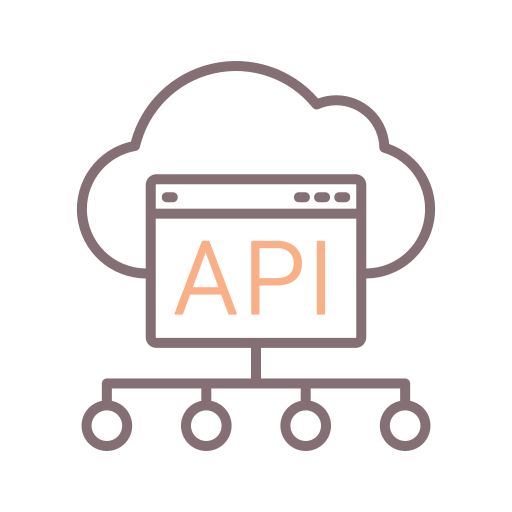
Optimizing Product Planning – Simplifying Cloud Cost Modeling using Azure Retail Pricing API
“Public Cloud providers’ online pricing calculators are so user friendly and easy to use that I love building a cost model for my enterprise SaaS Product” – Said NoOne
If you are building out a new SaaS service or planning to launch an existing service in a new geography it is most likely that among other things (services, features, latency compliance, and privacy) you would want to analyze the cost of hosting the SaaS service. Building out the cost model for a SaaS service may sound trivial but when you consider the price for different cloud services (compute, storage, databases network etc.), the variation of instance and tier types (archive, hot, cool, large, small etc.), different cloud regions, different cloud providers, and different savings plans (spot, on demand, and reserved etc.), it soon becomes unyielding. If you go about building the cost model using the online calculator provided by cloud providers it soon becomes a grunt work project. These online calculator tools are fine for simple models but not user friendly for building out enterprise SaaS. If for some reason you have to build the model multiple times the grunt work and the time sink become unbearable. As someone who often works in building V1.0 of complex enterprise SaaS services from scratch, building out a cost model is foundational. I have had my fair share of building the cost model using online calculators. The unproductivity of this manual work made me think about automating it and building a configurable tool to model quickly, accurately, and repeatedly without manual effort. This was my driver for exploring the retail pricing APIs from Azure and then I thought I may not be the only one with this problem so why not share my learnings so someone else may find it useful? With that context let’s dive in.
Azure Retail Pricing API overview:
Azure Retail Rates Prices APIs provide a programmatic way to retrieve retail prices for all Azure services. People can use these APIs to create tools for internal cost analysis and price comparison across SKUs and regions. Please keep in mind that these APIs are different from consumption or cost management APIs, using which an enterprise Azure customer can get price sheet, forecast, and usage details etc.
The good part is that these retail pricing APIs are not tied to a subscription, and therefore are not authenticated but if you are looking to build a tool for budget and spend tracking then please use cost management and consumption APIs to programmatically build that view.
If you want to learn more about these APIs check out these links for Azure documentation: Microsoft Azure Cost Management APIs, Microsoft Azure Consumption APIs, and Microsoft Azure Retail Price APIs.
Let’s dive deeper – The fun stuff:
Azure retail pricing APIs are easily filtered so you can fetch specific properties and the data you need.
Main properties and filters for API:
Below I am sharing details of selected properties and filters I found most useful, for a full list of properties and filters please use the link above for detailed API specifications.
Currency Code (currencyCode) provides details of the currency. The default is USD but the API supports more than 15 of the most common currencies across the globe for example EUR, GBP, INR, JPY etc..
Retail Price (retailPrice) provides the non discounted retail price. If your organization has an enterprise agreement with Azure and you want to know the price to you then please use the cost management and consumption APIs and not the retail APIs. Although I think retail pricing is still useful in some cases when you may want to compare the price of a service across different regions. The retail price will provide a directionally comparable view of different regions which is a good proxy for what one can expect after the discount price in the enterprise agreement.
Region Name (armRegionName) provides details of Azure Resource Manager region where the service is available for example southcentralus.
Location (location) provides details of Azure data center where the resource is deployed for example EU West.
Meter Name (meterName) the meter for billing.
Product Name (productName) name of the product.
Service Name (serviceName) name of the service. For example virtual machine etc.
Service Family (serviceFamily) name of the service family. For example, compute
Unit of Measure (unitOfMeasure) how the billing meter is measured. For example hour or GB etc.
Price Type (priceType) provides details of meter consumption type. For example consumption or reservation.
Example for Storage Service:
Example Program #1
This program queries the Azure Retail Price APIs and fetches price details for data stored in Azure cool blob, write operations, read operations, and data retrieval across the globe. The program saves the output as csv and json files on the computer.
#!/usr/bin/env python3
import requests
import json
from tabulate import tabulate
import pandas as pd
import os
import csv
def build_pricing_table(json_data, table_data):
for item in json_data["Items"]:
table_data.append(
[
item["armRegionName"],
item["location"],
item["meterName"],
item["type"],
item["serviceName"],
item["productName"],
item["currencyCode"],
item["retailPrice"],
item["unitOfMeasure"],
item["tierMinimumUnits"],
]
)
def main():
table_data = []
table_data.append(
[
"Region Name",
"Region",
"Meter",
"type",
"Service Name",
"Product Name",
"Currency Code",
"Retail Price",
"Unit of Measure",
"Minimum Units",
]
)
api_url = (
"https://prices.azure.com/api/retail/prices?api-version=2023-01-01-preview"
)
query = "productName eq 'General Block Blob v2 Hierarchical Namespace' and serviceName eq 'Storage' and (meterName eq 'Cool LRS Data Stored' or meterName eq 'Cool Write Operations' or meterName eq 'Cool Read Operations' or meterName eq 'Cool Data Retrieval')"
response = requests.get(api_url, params={"$filter": query})
json_data = json.loads(response.text)
build_pricing_table(json_data, table_data)
nextPage = json_data["NextPageLink"]
while nextPage:
response = requests.get(nextPage)
json_data = json.loads(response.text)
nextPage = json_data["NextPageLink"]
build_pricing_table(json_data, table_data)
# Print pricing data
print(tabulate(table_data, headers="firstrow", tablefmt="psql"))
# Set your file name to save the json to a csv file
csvfilename = "AzureCoolBlobPrice.csv"
jsonfilename = "AzureCoolBlobPrice.json"
# Save the price list data in json format to a data frame
with open(jsonfilename, "w", encoding="utf-8") as f:
json.dump(json_data, f, ensure_ascii=False, indent=4)
# save the price list data in a csv file
price_df = pd.DataFrame(table_data)
price_df.to_csv(csvfilename, index=False, header=False)
if __name__ == "__main__":
main()
Output:
CSV File:
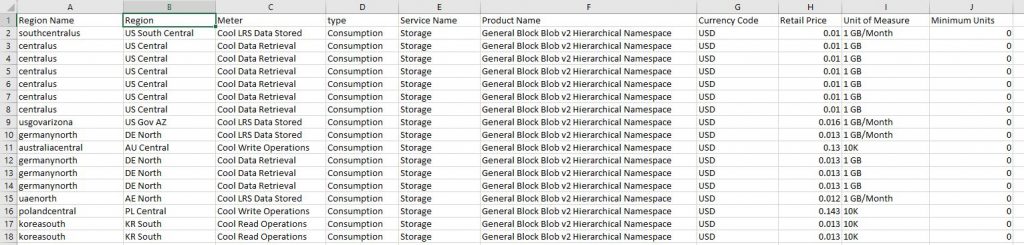
JSON File:
{
"BillingCurrency": "USD",
"CustomerEntityId": "Default",
"CustomerEntityType": "Retail",
"Items": [
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"retailPrice": 0.01,
"unitPrice": 0.01,
"armRegionName": "northeurope",
"location": "EU North",
"effectiveStartDate": "2020-03-01T00:00:00Z",
"meterId": "f1f955a9-50ca-493c-8bd3-f6c7e3ab5c4a",
"meterName": "Cool LRS Data Stored",
"productId": "DZH318Z0C120",
"skuId": "DZH318Z0C120/005R",
"productName": "General Block Blob v2 Hierarchical Namespace",
"skuName": "Cool LRS",
"serviceName": "Storage",
"serviceId": "DZH317F1HKN0",
"serviceFamily": "Storage",
"unitOfMeasure": "1 GB/Month",
"type": "Consumption",
"isPrimaryMeterRegion": true,
"armSkuName": ""
},
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"retailPrice": 0.013,
"unitPrice": 0.013,
"armRegionName": "japanwest",
"location": "JA West",
"effectiveStartDate": "2020-03-01T00:00:00Z",
"meterId": "f246c2dd-c573-4672-945b-60f6edb2f248",
"meterName": "Cool Read Operations",
"productId": "DZH318Z0C120",
"skuId": "DZH318Z0C120/008T",
"productName": "General Block Blob v2 Hierarchical Namespace",
"skuName": "Cool GRS",
"serviceName": "Storage",
"serviceId": "DZH317F1HKN0",
"serviceFamily": "Storage",
"unitOfMeasure": "10K",
"type": "Consumption",
"isPrimaryMeterRegion": false,
"armSkuName": ""
},
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"retailPrice": 0.013,
"unitPrice": 0.013,
"armRegionName": "japanwest",
"location": "JA West",
"effectiveStartDate": "2020-03-01T00:00:00Z",
"meterId": "f246c2dd-c573-4672-945b-60f6edb2f248",
"meterName": "Cool Read Operations",
"productId": "DZH318Z0C120",
"skuId": "DZH318Z0C120/00C8",
"productName": "General Block Blob v2 Hierarchical Namespace",
"skuName": "Cool RA-GRS",
"serviceName": "Storage",
"serviceId": "DZH317F1HKN0",
"serviceFamily": "Storage",
"unitOfMeasure": "10K",
"type": "Consumption",
"isPrimaryMeterRegion": false,
"armSkuName": ""
},
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"retailPrice": 0.013,
"unitPrice": 0.013,
"armRegionName": "japanwest",
"location": "JA West",
"effectiveStartDate": "2020-03-01T00:00:00Z",
"meterId": "f246c2dd-c573-4672-945b-60f6edb2f248",
"meterName": "Cool Read Operations",
"productId": "DZH318Z0C120",
"skuId": "DZH318Z0C120/008P",
"productName": "General Block Blob v2 Hierarchical Namespace",
"skuName": "Cool LRS",
"serviceName": "Storage",
"serviceId": "DZH317F1HKN0",
"serviceFamily": "Storage",
"unitOfMeasure": "10K",
"type": "Consumption",
"isPrimaryMeterRegion": true,
"armSkuName": ""
},
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"retailPrice": 0.011,
"unitPrice": 0.011,
"armRegionName": "canadacentral",
"location": "CA Central",
"effectiveStartDate": "2020-03-01T00:00:00Z",
"meterId": "f28a82af-a135-4481-8a73-149d07951b0b",
"meterName": "Cool LRS Data Stored",
"productId": "DZH318Z0C120",
"skuId": "DZH318Z0C120/006G",
"productName": "General Block Blob v2 Hierarchical Namespace",
"skuName": "Cool LRS",
"serviceName": "Storage",
"serviceId": "DZH317F1HKN0",
"serviceFamily": "Storage",
"unitOfMeasure": "1 GB/Month",
"type": "Consumption",
"isPrimaryMeterRegion": true,
"armSkuName": ""
},
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"retailPrice": 0.015,
"unitPrice": 0.015,
"armRegionName": "australiacentral",
"location": "AU Central",
"effectiveStartDate": "2020-03-01T00:00:00Z",
"meterId": "f46cbd0e-ae72-423d-a983-dba52e5aac3c",
"meterName": "Cool LRS Data Stored",
"productId": "DZH318Z0C120",
"skuId": "DZH318Z0C120/0070",
"productName": "General Block Blob v2 Hierarchical Namespace",
"skuName": "Cool LRS",
"serviceName": "Storage",
"serviceId": "DZH317F1HKN0",
"serviceFamily": "Storage",
"unitOfMeasure": "1 GB/Month",
"type": "Consumption",
"isPrimaryMeterRegion": true,
"armSkuName": ""
},
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"retailPrice": 0.143,
"unitPrice": 0.143,
"armRegionName": "norwayeast",
"location": "NO East",
"effectiveStartDate": "2020-01-23T00:00:00Z",
"meterId": "f4923fea-f2e3-454e-b555-c56ff3417bf5",
"meterName": "Cool Write Operations",
"productId": "DZH318Z0C120",
"skuId": "DZH318Z0C120/00K1",
"productName": "General Block Blob v2 Hierarchical Namespace",
"skuName": "Cool LRS",
"serviceName": "Storage",
"serviceId": "DZH317F1HKN0",
"serviceFamily": "Storage",
"unitOfMeasure": "10K",
"type": "Consumption",
"isPrimaryMeterRegion": true,
"armSkuName": ""
},
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"retailPrice": 0.0143,
"unitPrice": 0.0143,
"armRegionName": "switzerlandnorth",
"location": "CH North",
"effectiveStartDate": "2021-10-01T00:00:00Z",
"meterId": "f5f7b7de-f04a-4914-ad99-22a18cce0f06",
"meterName": "Cool Read Operations",
"productId": "DZH318Z0C120",
"skuId": "DZH318Z0C120/00DK",
"productName": "General Block Blob v2 Hierarchical Namespace",
"skuName": "Cool GRS",
"serviceName": "Storage",
"serviceId": "DZH317F1HKN0",
"serviceFamily": "Storage",
"unitOfMeasure": "10K",
"type": "Consumption",
"isPrimaryMeterRegion": false,
"armSkuName": ""
},
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"retailPrice": 0.0143,
"unitPrice": 0.0143,
"armRegionName": "switzerlandnorth",
"location": "CH North",
"effectiveStartDate": "2021-10-01T00:00:00Z",
"meterId": "f5f7b7de-f04a-4914-ad99-22a18cce0f06",
"meterName": "Cool Read Operations",
"productId": "DZH318Z0C120",
"skuId": "DZH318Z0C120/00DL",
"productName": "General Block Blob v2 Hierarchical Namespace",
"skuName": "Cool RA-GRS",
"serviceName": "Storage",
"serviceId": "DZH317F1HKN0",
"serviceFamily": "Storage",
"unitOfMeasure": "10K",
"type": "Consumption",
"isPrimaryMeterRegion": false,
"armSkuName": ""
},
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"retailPrice": 0.0143,
"unitPrice": 0.0143,
"armRegionName": "switzerlandnorth",
"location": "CH North",
"effectiveStartDate": "2021-10-01T00:00:00Z",
"meterId": "f5f7b7de-f04a-4914-ad99-22a18cce0f06",
"meterName": "Cool Read Operations",
"productId": "DZH318Z0C120",
"skuId": "DZH318Z0C120/00DN",
"productName": "General Block Blob v2 Hierarchical Namespace",
"skuName": "Cool LRS",
"serviceName": "Storage",
"serviceId": "DZH317F1HKN0",
"serviceFamily": "Storage",
"unitOfMeasure": "10K",
"type": "Consumption",
"isPrimaryMeterRegion": true,
"armSkuName": ""
},
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"retailPrice": 0.01,
"unitPrice": 0.01,
"armRegionName": "eastus2",
"location": "US East 2",
"effectiveStartDate": "2020-03-01T00:00:00Z",
"meterId": "f7caf658-b1e9-4247-ace8-22220d86435e",
"meterName": "Cool Data Retrieval",
"productId": "DZH318Z0C120",
"skuId": "DZH318Z0C120/004H",
"productName": "General Block Blob v2 Hierarchical Namespace",
"skuName": "Cool LRS",
"serviceName": "Storage",
"serviceId": "DZH317F1HKN0",
"serviceFamily": "Storage",
"unitOfMeasure": "1 GB",
"type": "Consumption",
"isPrimaryMeterRegion": true,
"armSkuName": ""
},
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"retailPrice": 0.01,
"unitPrice": 0.01,
"armRegionName": "eastus2",
"location": "US East 2",
"effectiveStartDate": "2020-03-01T00:00:00Z",
"meterId": "f7caf658-b1e9-4247-ace8-22220d86435e",
"meterName": "Cool Data Retrieval",
"productId": "DZH318Z0C120",
"skuId": "DZH318Z0C120/004C",
"productName": "General Block Blob v2 Hierarchical Namespace",
"skuName": "Cool GRS",
"serviceName": "Storage",
"serviceId": "DZH317F1HKN0",
"serviceFamily": "Storage",
"unitOfMeasure": "1 GB",
"type": "Consumption",
"isPrimaryMeterRegion": false,
"armSkuName": ""
},
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"retailPrice": 0.01,
"unitPrice": 0.01,
"armRegionName": "eastus2",
"location": "US East 2",
"effectiveStartDate": "2020-03-01T00:00:00Z",
"meterId": "f7caf658-b1e9-4247-ace8-22220d86435e",
"meterName": "Cool Data Retrieval",
"productId": "DZH318Z0C120",
"skuId": "DZH318Z0C120/00BQ",
"productName": "General Block Blob v2 Hierarchical Namespace",
"skuName": "Cool RA-GRS",
"serviceName": "Storage",
"serviceId": "DZH317F1HKN0",
"serviceFamily": "Storage",
"unitOfMeasure": "1 GB",
"type": "Consumption",
"isPrimaryMeterRegion": false,
"armSkuName": ""
},
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"retailPrice": 0.01,
"unitPrice": 0.01,
"armRegionName": "eastus2",
"location": "US East 2",
"effectiveStartDate": "2020-03-01T00:00:00Z",
"meterId": "f7caf658-b1e9-4247-ace8-22220d86435e",
"meterName": "Cool Data Retrieval",
"productId": "DZH318Z0C120",
"skuId": "DZH318Z0C120/00G9",
"productName": "General Block Blob v2 Hierarchical Namespace",
"skuName": "Cool RA-GZRS",
"serviceName": "Storage",
"serviceId": "DZH317F1HKN0",
"serviceFamily": "Storage",
"unitOfMeasure": "1 GB",
"type": "Consumption",
"isPrimaryMeterRegion": false,
"armSkuName": ""
},
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"retailPrice": 0.01,
"unitPrice": 0.01,
"armRegionName": "eastus2",
"location": "US East 2",
"effectiveStartDate": "2020-03-01T00:00:00Z",
"meterId": "f7caf658-b1e9-4247-ace8-22220d86435e",
"meterName": "Cool Data Retrieval",
"productId": "DZH318Z0C120",
"skuId": "DZH318Z0C120/00G8",
"productName": "General Block Blob v2 Hierarchical Namespace",
"skuName": "Cool GZRS",
"serviceName": "Storage",
"serviceId": "DZH317F1HKN0",
"serviceFamily": "Storage",
"unitOfMeasure": "1 GB",
"type": "Consumption",
"isPrimaryMeterRegion": false,
"armSkuName": ""
},
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"retailPrice": 0.01,
"unitPrice": 0.01,
"armRegionName": "eastus2",
"location": "US East 2",
"effectiveStartDate": "2020-03-01T00:00:00Z",
"meterId": "f7caf658-b1e9-4247-ace8-22220d86435e",
"meterName": "Cool Data Retrieval",
"productId": "DZH318Z0C120",
"skuId": "DZH318Z0C120/004K",
"productName": "General Block Blob v2 Hierarchical Namespace",
"skuName": "Cool ZRS",
"serviceName": "Storage",
"serviceId": "DZH317F1HKN0",
"serviceFamily": "Storage",
"unitOfMeasure": "1 GB",
"type": "Consumption",
"isPrimaryMeterRegion": false,
"armSkuName": ""
},
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"retailPrice": 0.01,
"unitPrice": 0.01,
"armRegionName": "centralindia",
"location": "IN Central",
"effectiveStartDate": "2020-03-01T00:00:00Z",
"meterId": "f7ee9ff7-3297-4134-970f-14cfd6e5af0e",
"meterName": "Cool Data Retrieval",
"productId": "DZH318Z0C120",
"skuId": "DZH318Z0C120/00CR",
"productName": "General Block Blob v2 Hierarchical Namespace",
"skuName": "Cool RA-GRS",
"serviceName": "Storage",
"serviceId": "DZH317F1HKN0",
"serviceFamily": "Storage",
"unitOfMeasure": "1 GB",
"type": "Consumption",
"isPrimaryMeterRegion": false,
"armSkuName": ""
},
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"retailPrice": 0.01,
"unitPrice": 0.01,
"armRegionName": "centralindia",
"location": "IN Central",
"effectiveStartDate": "2020-03-01T00:00:00Z",
"meterId": "f7ee9ff7-3297-4134-970f-14cfd6e5af0e",
"meterName": "Cool Data Retrieval",
"productId": "DZH318Z0C120",
"skuId": "DZH318Z0C120/009P",
"productName": "General Block Blob v2 Hierarchical Namespace",
"skuName": "Cool LRS",
"serviceName": "Storage",
"serviceId": "DZH317F1HKN0",
"serviceFamily": "Storage",
"unitOfMeasure": "1 GB",
"type": "Consumption",
"isPrimaryMeterRegion": true,
"armSkuName": ""
},
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"retailPrice": 0.01,
"unitPrice": 0.01,
"armRegionName": "centralindia",
"location": "IN Central",
"effectiveStartDate": "2020-03-01T00:00:00Z",
"meterId": "f7ee9ff7-3297-4134-970f-14cfd6e5af0e",
"meterName": "Cool Data Retrieval",
"productId": "DZH318Z0C120",
"skuId": "DZH318Z0C120/009Q",
"productName": "General Block Blob v2 Hierarchical Namespace",
"skuName": "Cool ZRS",
"serviceName": "Storage",
"serviceId": "DZH317F1HKN0",
"serviceFamily": "Storage",
"unitOfMeasure": "1 GB",
"type": "Consumption",
"isPrimaryMeterRegion": false,
"armSkuName": ""
},
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"retailPrice": 0.01,
"unitPrice": 0.01,
"armRegionName": "centralindia",
"location": "IN Central",
"effectiveStartDate": "2020-03-01T00:00:00Z",
"meterId": "f7ee9ff7-3297-4134-970f-14cfd6e5af0e",
"meterName": "Cool Data Retrieval",
"productId": "DZH318Z0C120",
"skuId": "DZH318Z0C120/009M",
"productName": "General Block Blob v2 Hierarchical Namespace",
"skuName": "Cool GRS",
"serviceName": "Storage",
"serviceId": "DZH317F1HKN0",
"serviceFamily": "Storage",
"unitOfMeasure": "1 GB",
"type": "Consumption",
"isPrimaryMeterRegion": false,
"armSkuName": ""
},
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"retailPrice": 0.13,
"unitPrice": 0.13,
"armRegionName": "westus2",
"location": "US West 2",
"effectiveStartDate": "2020-03-01T00:00:00Z",
"meterId": "f891bc05-10c3-46f3-a473-e2cfd7751aed",
"meterName": "Cool Write Operations",
"productId": "DZH318Z0C120",
"skuId": "DZH318Z0C120/002J",
"productName": "General Block Blob v2 Hierarchical Namespace",
"skuName": "Cool LRS",
"serviceName": "Storage",
"serviceId": "DZH317F1HKN0",
"serviceFamily": "Storage",
"unitOfMeasure": "10K",
"type": "Consumption",
"isPrimaryMeterRegion": true,
"armSkuName": ""
},
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"retailPrice": 0.0105,
"unitPrice": 0.0105,
"armRegionName": "italynorth",
"location": "IT North",
"effectiveStartDate": "2023-06-27T00:00:00Z",
"meterId": "fb197c78-9877-5e45-bf9e-7fea5b504e37",
"meterName": "Cool LRS Data Stored",
"productId": "DZH318Z0C120",
"skuId": "DZH318Z0C120/0163",
"productName": "General Block Blob v2 Hierarchical Namespace",
"skuName": "Cool LRS",
"serviceName": "Storage",
"serviceId": "DZH317F1HKN0",
"serviceFamily": "Storage",
"unitOfMeasure": "1 GB/Month",
"type": "Consumption",
"isPrimaryMeterRegion": true,
"armSkuName": ""
},
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"retailPrice": 0.143,
"unitPrice": 0.143,
"armRegionName": "switzerlandnorth",
"location": "CH North",
"effectiveStartDate": "2021-10-01T00:00:00Z",
"meterId": "fcecca5a-1852-4a9e-90b4-1894ddea2ede",
"meterName": "Cool Write Operations",
"productId": "DZH318Z0C120",
"skuId": "DZH318Z0C120/00DN",
"productName": "General Block Blob v2 Hierarchical Namespace",
"skuName": "Cool LRS",
"serviceName": "Storage",
"serviceId": "DZH317F1HKN0",
"serviceFamily": "Storage",
"unitOfMeasure": "10K",
"type": "Consumption",
"isPrimaryMeterRegion": true,
"armSkuName": ""
},
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"retailPrice": 0.13,
"unitPrice": 0.13,
"armRegionName": "australiacentral2",
"location": "AU Central 2",
"effectiveStartDate": "2020-03-01T00:00:00Z",
"meterId": "fddab211-47b8-4a1d-aae7-f47658894ed1",
"meterName": "Cool Write Operations",
"productId": "DZH318Z0C120",
"skuId": "DZH318Z0C120/005T",
"productName": "General Block Blob v2 Hierarchical Namespace",
"skuName": "Cool LRS",
"serviceName": "Storage",
"serviceId": "DZH317F1HKN0",
"serviceFamily": "Storage",
"unitOfMeasure": "10K",
"type": "Consumption",
"isPrimaryMeterRegion": true,
"armSkuName": ""
},
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"retailPrice": 0.0177,
"unitPrice": 0.0177,
"armRegionName": "brazilsouth",
"location": "BR South",
"effectiveStartDate": "2022-02-01T00:00:00Z",
"meterId": "fe3554bd-c798-4e69-83a0-4b57fabbe9ff",
"meterName": "Cool LRS Data Stored",
"productId": "DZH318Z0C120",
"skuId": "DZH318Z0C120/0059",
"productName": "General Block Blob v2 Hierarchical Namespace",
"skuName": "Cool LRS",
"serviceName": "Storage",
"serviceId": "DZH317F1HKN0",
"serviceFamily": "Storage",
"unitOfMeasure": "1 GB/Month",
"type": "Consumption",
"isPrimaryMeterRegion": true,
"armSkuName": ""
}
],
"NextPageLink": null,
"Count": 25
}
Example Program #2:
This program queries the Azure Retail Price APIs and fetches price details for data stored in Azure Archive blob, write operations, read operations, priority data retrieval and data retrieval for US South Central region. The program saves the output as csv and json files on the computer.
#!/usr/bin/env python3
import requests
import json
from tabulate import tabulate
import pandas as pd
import os
import csv
def build_pricing_table(json_data, table_data):
for item in json_data["Items"]:
table_data.append(
[
item["armRegionName"],
item["location"],
item["meterName"],
item["type"],
item["serviceName"],
item["productName"],
item["currencyCode"],
item["retailPrice"],
item["unitOfMeasure"],
]
)
def main():
table_data = []
table_data.append(
[
"Region Name",
"Region",
"Meter",
"type",
"Service Name",
"Product Name",
"Currency Code",
"Retail Price",
"Unit of Measure",
]
)
api_url = (
"https://prices.azure.com/api/retail/prices?api-version=2023-01-01-preview"
)
query = "armRegionName eq 'southcentralus' and productName eq 'General Block Blob v2 Hierarchical Namespace' and serviceName eq 'Storage' and (meterName eq 'Archive LRS Data Stored' or meterName eq 'Archive GRS Data Stored' or meterName eq 'Archive Read Operations' or meterName eq 'Archive Write Operations' or meterName eq 'Archive Data Retrieval' or meterName eq 'Archive Priority Data Retrieval')"
response = requests.get(api_url, params={"$filter": query})
json_data = json.loads(response.text)
build_pricing_table(json_data, table_data)
nextPage = json_data["NextPageLink"]
while nextPage:
response = requests.get(nextPage)
json_data = json.loads(response.text)
nextPage = json_data["NextPageLink"]
build_pricing_table(json_data, table_data)
# Print pricing data
print(tabulate(table_data, headers="firstrow", tablefmt="psql"))
# Set your file name to save the json to a csv file
csvfilename = "AzureArchiveBlobPrice.csv"
jsonfilename = "AzureArchiveBlobPrice.json"
# Save the price list data in json format to a data frame
with open(jsonfilename, "w", encoding="utf-8") as f:
json.dump(json_data, f, ensure_ascii=False, indent=4)
# save the price list data in a csv file
price_df = pd.DataFrame(table_data)
price_df.to_csv(csvfilename, index=False, header=False)
if __name__ == "__main__":
main()
Output:
CSV file:
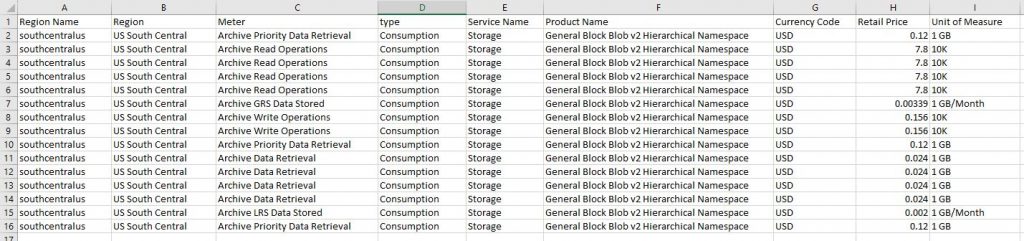
JSON file:
{
"BillingCurrency": "USD",
"CustomerEntityId": "Default",
"CustomerEntityType": "Retail",
"Items": [
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"retailPrice": 0.12,
"unitPrice": 0.12,
"armRegionName": "southcentralus",
"location": "US South Central",
"effectiveStartDate": "2022-12-01T00:00:00Z",
"meterId": "3a266598-6bc2-50a7-8be6-7dc411a14229",
"meterName": "Archive Priority Data Retrieval",
"productId": "DZH318Z0C120",
"skuId": "DZH318Z0C120/00BG",
"productName": "General Block Blob v2 Hierarchical Namespace",
"skuName": "Archive RA-GRS",
"serviceName": "Storage",
"serviceId": "DZH317F1HKN0",
"serviceFamily": "Storage",
"unitOfMeasure": "1 GB",
"type": "Consumption",
"isPrimaryMeterRegion": true,
"armSkuName": ""
},
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"retailPrice": 7.8,
"unitPrice": 7.8,
"armRegionName": "southcentralus",
"location": "US South Central",
"effectiveStartDate": "2020-03-01T00:00:00Z",
"meterId": "6648f174-58d6-475f-b761-668603d65bc7",
"meterName": "Archive Read Operations",
"productId": "DZH318Z0C120",
"skuId": "DZH318Z0C120/0032",
"productName": "General Block Blob v2 Hierarchical Namespace",
"skuName": "Archive GRS",
"serviceName": "Storage",
"serviceId": "DZH317F1HKN0",
"serviceFamily": "Storage",
"unitOfMeasure": "10K",
"type": "Consumption",
"isPrimaryMeterRegion": false,
"armSkuName": ""
},
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"retailPrice": 7.8,
"unitPrice": 7.8,
"armRegionName": "southcentralus",
"location": "US South Central",
"effectiveStartDate": "2020-03-01T00:00:00Z",
"meterId": "6648f174-58d6-475f-b761-668603d65bc7",
"meterName": "Archive Read Operations",
"productId": "DZH318Z0C120",
"skuId": "DZH318Z0C120/00KF",
"productName": "General Block Blob v2 Hierarchical Namespace",
"skuName": "Archive ZRS",
"serviceName": "Storage",
"serviceId": "DZH317F1HKN0",
"serviceFamily": "Storage",
"unitOfMeasure": "10K",
"type": "Consumption",
"isPrimaryMeterRegion": false,
"armSkuName": ""
},
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"retailPrice": 7.8,
"unitPrice": 7.8,
"armRegionName": "southcentralus",
"location": "US South Central",
"effectiveStartDate": "2020-03-01T00:00:00Z",
"meterId": "6648f174-58d6-475f-b761-668603d65bc7",
"meterName": "Archive Read Operations",
"productId": "DZH318Z0C120",
"skuId": "DZH318Z0C120/00BG",
"productName": "General Block Blob v2 Hierarchical Namespace",
"skuName": "Archive RA-GRS",
"serviceName": "Storage",
"serviceId": "DZH317F1HKN0",
"serviceFamily": "Storage",
"unitOfMeasure": "10K",
"type": "Consumption",
"isPrimaryMeterRegion": false,
"armSkuName": ""
},
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"retailPrice": 7.8,
"unitPrice": 7.8,
"armRegionName": "southcentralus",
"location": "US South Central",
"effectiveStartDate": "2020-03-01T00:00:00Z",
"meterId": "6648f174-58d6-475f-b761-668603d65bc7",
"meterName": "Archive Read Operations",
"productId": "DZH318Z0C120",
"skuId": "DZH318Z0C120/003G",
"productName": "General Block Blob v2 Hierarchical Namespace",
"skuName": "Archive LRS",
"serviceName": "Storage",
"serviceId": "DZH317F1HKN0",
"serviceFamily": "Storage",
"unitOfMeasure": "10K",
"type": "Consumption",
"isPrimaryMeterRegion": true,
"armSkuName": ""
},
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"retailPrice": 0.00339,
"unitPrice": 0.00339,
"armRegionName": "southcentralus",
"location": "US South Central",
"effectiveStartDate": "2020-11-01T00:00:00Z",
"meterId": "9569d93d-853b-4fd0-b3e4-7f9bacef0026",
"meterName": "Archive GRS Data Stored",
"productId": "DZH318Z0C120",
"skuId": "DZH318Z0C120/0032",
"productName": "General Block Blob v2 Hierarchical Namespace",
"skuName": "Archive GRS",
"serviceName": "Storage",
"serviceId": "DZH317F1HKN0",
"serviceFamily": "Storage",
"unitOfMeasure": "1 GB/Month",
"type": "Consumption",
"isPrimaryMeterRegion": true,
"armSkuName": ""
},
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"retailPrice": 0.156,
"unitPrice": 0.156,
"armRegionName": "southcentralus",
"location": "US South Central",
"effectiveStartDate": "2020-03-01T00:00:00Z",
"meterId": "b4bcb456-77c8-446d-a78c-6e2cfba1dee7",
"meterName": "Archive Write Operations",
"productId": "DZH318Z0C120",
"skuId": "DZH318Z0C120/00KF",
"productName": "General Block Blob v2 Hierarchical Namespace",
"skuName": "Archive ZRS",
"serviceName": "Storage",
"serviceId": "DZH317F1HKN0",
"serviceFamily": "Storage",
"unitOfMeasure": "10K",
"type": "Consumption",
"isPrimaryMeterRegion": false,
"armSkuName": ""
},
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"retailPrice": 0.156,
"unitPrice": 0.156,
"armRegionName": "southcentralus",
"location": "US South Central",
"effectiveStartDate": "2020-03-01T00:00:00Z",
"meterId": "b4bcb456-77c8-446d-a78c-6e2cfba1dee7",
"meterName": "Archive Write Operations",
"productId": "DZH318Z0C120",
"skuId": "DZH318Z0C120/003G",
"productName": "General Block Blob v2 Hierarchical Namespace",
"skuName": "Archive LRS",
"serviceName": "Storage",
"serviceId": "DZH317F1HKN0",
"serviceFamily": "Storage",
"unitOfMeasure": "10K",
"type": "Consumption",
"isPrimaryMeterRegion": true,
"armSkuName": ""
},
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"retailPrice": 0.12,
"unitPrice": 0.12,
"armRegionName": "southcentralus",
"location": "US South Central",
"effectiveStartDate": "2022-12-01T00:00:00Z",
"meterId": "b4ea44d4-fcf7-5e92-b0ae-cb24c46d65f5",
"meterName": "Archive Priority Data Retrieval",
"productId": "DZH318Z0C120",
"skuId": "DZH318Z0C120/0032",
"productName": "General Block Blob v2 Hierarchical Namespace",
"skuName": "Archive GRS",
"serviceName": "Storage",
"serviceId": "DZH317F1HKN0",
"serviceFamily": "Storage",
"unitOfMeasure": "1 GB",
"type": "Consumption",
"isPrimaryMeterRegion": true,
"armSkuName": ""
},
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"retailPrice": 0.024,
"unitPrice": 0.024,
"armRegionName": "southcentralus",
"location": "US South Central",
"effectiveStartDate": "2020-03-01T00:00:00Z",
"meterId": "e2c8d96c-59f0-4bf8-9b11-b2ce383d7752",
"meterName": "Archive Data Retrieval",
"productId": "DZH318Z0C120",
"skuId": "DZH318Z0C120/003G",
"productName": "General Block Blob v2 Hierarchical Namespace",
"skuName": "Archive LRS",
"serviceName": "Storage",
"serviceId": "DZH317F1HKN0",
"serviceFamily": "Storage",
"unitOfMeasure": "1 GB",
"type": "Consumption",
"isPrimaryMeterRegion": true,
"armSkuName": ""
},
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"retailPrice": 0.024,
"unitPrice": 0.024,
"armRegionName": "southcentralus",
"location": "US South Central",
"effectiveStartDate": "2020-03-01T00:00:00Z",
"meterId": "e2c8d96c-59f0-4bf8-9b11-b2ce383d7752",
"meterName": "Archive Data Retrieval",
"productId": "DZH318Z0C120",
"skuId": "DZH318Z0C120/00BG",
"productName": "General Block Blob v2 Hierarchical Namespace",
"skuName": "Archive RA-GRS",
"serviceName": "Storage",
"serviceId": "DZH317F1HKN0",
"serviceFamily": "Storage",
"unitOfMeasure": "1 GB",
"type": "Consumption",
"isPrimaryMeterRegion": false,
"armSkuName": ""
},
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"retailPrice": 0.024,
"unitPrice": 0.024,
"armRegionName": "southcentralus",
"location": "US South Central",
"effectiveStartDate": "2020-03-01T00:00:00Z",
"meterId": "e2c8d96c-59f0-4bf8-9b11-b2ce383d7752",
"meterName": "Archive Data Retrieval",
"productId": "DZH318Z0C120",
"skuId": "DZH318Z0C120/00KF",
"productName": "General Block Blob v2 Hierarchical Namespace",
"skuName": "Archive ZRS",
"serviceName": "Storage",
"serviceId": "DZH317F1HKN0",
"serviceFamily": "Storage",
"unitOfMeasure": "1 GB",
"type": "Consumption",
"isPrimaryMeterRegion": false,
"armSkuName": ""
},
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"retailPrice": 0.024,
"unitPrice": 0.024,
"armRegionName": "southcentralus",
"location": "US South Central",
"effectiveStartDate": "2020-03-01T00:00:00Z",
"meterId": "e2c8d96c-59f0-4bf8-9b11-b2ce383d7752",
"meterName": "Archive Data Retrieval",
"productId": "DZH318Z0C120",
"skuId": "DZH318Z0C120/0032",
"productName": "General Block Blob v2 Hierarchical Namespace",
"skuName": "Archive GRS",
"serviceName": "Storage",
"serviceId": "DZH317F1HKN0",
"serviceFamily": "Storage",
"unitOfMeasure": "1 GB",
"type": "Consumption",
"isPrimaryMeterRegion": false,
"armSkuName": ""
},
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"retailPrice": 0.002,
"unitPrice": 0.002,
"armRegionName": "southcentralus",
"location": "US South Central",
"effectiveStartDate": "2020-03-01T00:00:00Z",
"meterId": "e3319b6a-a594-4665-be2b-7f9a97fc6a7b",
"meterName": "Archive LRS Data Stored",
"productId": "DZH318Z0C120",
"skuId": "DZH318Z0C120/003G",
"productName": "General Block Blob v2 Hierarchical Namespace",
"skuName": "Archive LRS",
"serviceName": "Storage",
"serviceId": "DZH317F1HKN0",
"serviceFamily": "Storage",
"unitOfMeasure": "1 GB/Month",
"type": "Consumption",
"isPrimaryMeterRegion": true,
"armSkuName": ""
},
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"retailPrice": 0.12,
"unitPrice": 0.12,
"armRegionName": "southcentralus",
"location": "US South Central",
"effectiveStartDate": "2022-12-01T00:00:00Z",
"meterId": "e5ac91c7-53d8-584a-a7a4-f3cccf766696",
"meterName": "Archive Priority Data Retrieval",
"productId": "DZH318Z0C120",
"skuId": "DZH318Z0C120/003G",
"productName": "General Block Blob v2 Hierarchical Namespace",
"skuName": "Archive LRS",
"serviceName": "Storage",
"serviceId": "DZH317F1HKN0",
"serviceFamily": "Storage",
"unitOfMeasure": "1 GB",
"type": "Consumption",
"isPrimaryMeterRegion": true,
"armSkuName": ""
}
],
"NextPageLink": null,
"Count": 15
}
Example for Compute Service:
Example Program #3
This program queries the Azure Retail Price APIs and fetches the price for reserved instance of Edv5 Series Azure VM in the US West region. The program saves the output a csv and json files on the computer.
#!/usr/bin/env python3
import requests
import json
from tabulate import tabulate
import pandas as pd
import os
import csv
def build_pricing_table(json_data, table_data):
for item in json_data["Items"]:
table_data.append(
[
item["armRegionName"],
item["location"],
item["meterName"],
item["serviceName"],
item["productName"],
item["skuName"],
item["currencyCode"],
item["retailPrice"],
item["type"],
item["reservationTerm"],
]
)
def main():
table_data = []
table_data.append(
[
"Region Name",
"Region",
"Meter",
"Service Name",
"Product Name",
"SKU Name",
"Currency Code",
"Retail Price",
"Price Type",
"Reservation Term",
]
)
api_url = (
"https://prices.azure.com/api/retail/prices?api-version=2023-01-01-preview"
)
query = "armRegionName eq 'westus' and serviceName eq 'Virtual Machines' and serviceFamily eq 'Compute' and productName eq 'Virtual Machines Edv5 Series' and priceType eq 'Reservation' "
response = requests.get(api_url, params={"$filter": query})
json_data = json.loads(response.text)
build_pricing_table(json_data, table_data)
nextPage = json_data["NextPageLink"]
while nextPage:
response = requests.get(nextPage)
json_data = json.loads(response.text)
nextPage = json_data["NextPageLink"]
build_pricing_table(json_data, table_data)
# Print pricing data
print(tabulate(table_data, headers="firstrow", tablefmt="psql"))
# Set your file name to save the json to a csv file
csvfilename = "AzureComputePrice.csv"
jsonfilename = "AzureComputePrice.json"
# Save the price list data in json format to a data frame
with open(jsonfilename, "w", encoding="utf-8") as f:
json.dump(json_data, f, ensure_ascii=False, indent=4)
# save the price list data in a csv file
price_df = pd.DataFrame(table_data)
price_df.to_csv(csvfilename, index=False, header=False)
if __name__ == "__main__":
main()
Output:
CSV file:
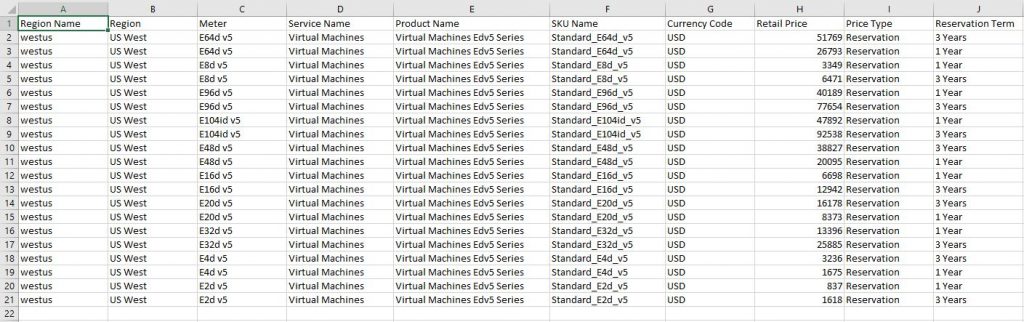
JSON file:
{
"BillingCurrency": "USD",
"CustomerEntityId": "Default",
"CustomerEntityType": "Retail",
"Items": [
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"reservationTerm": "3 Years",
"retailPrice": 51769.0,
"unitPrice": 51769.0,
"armRegionName": "westus",
"location": "US West",
"effectiveStartDate": "2021-11-01T00:00:00Z",
"meterId": "6a04be2a-08aa-5ba5-8035-488239d201df",
"meterName": "E64d v5",
"productId": "DZH318Z08M9R",
"skuId": "DZH318Z08M9R/021F",
"productName": "Virtual Machines Edv5 Series",
"skuName": "Standard_E64d_v5",
"serviceName": "Virtual Machines",
"serviceId": "DZH313Z7MMC8",
"serviceFamily": "Compute",
"unitOfMeasure": "1 Hour",
"type": "Reservation",
"isPrimaryMeterRegion": true,
"armSkuName": "Standard_E64d_v5"
},
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"reservationTerm": "1 Year",
"retailPrice": 26793.0,
"unitPrice": 26793.0,
"armRegionName": "westus",
"location": "US West",
"effectiveStartDate": "2021-11-01T00:00:00Z",
"meterId": "6a04be2a-08aa-5ba5-8035-488239d201df",
"meterName": "E64d v5",
"productId": "DZH318Z08M9R",
"skuId": "DZH318Z08M9R/021M",
"productName": "Virtual Machines Edv5 Series",
"skuName": "Standard_E64d_v5",
"serviceName": "Virtual Machines",
"serviceId": "DZH313Z7MMC8",
"serviceFamily": "Compute",
"unitOfMeasure": "1 Hour",
"type": "Reservation",
"isPrimaryMeterRegion": true,
"armSkuName": "Standard_E64d_v5"
},
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"reservationTerm": "1 Year",
"retailPrice": 3349.0,
"unitPrice": 3349.0,
"armRegionName": "westus",
"location": "US West",
"effectiveStartDate": "2021-11-01T00:00:00Z",
"meterId": "9259894d-0c3c-5b5b-a8be-c1de562f0fb0",
"meterName": "E8d v5",
"productId": "DZH318Z08M9R",
"skuId": "DZH318Z08M9R/022B",
"productName": "Virtual Machines Edv5 Series",
"skuName": "Standard_E8d_v5",
"serviceName": "Virtual Machines",
"serviceId": "DZH313Z7MMC8",
"serviceFamily": "Compute",
"unitOfMeasure": "1 Hour",
"type": "Reservation",
"isPrimaryMeterRegion": true,
"armSkuName": "Standard_E8d_v5"
},
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"reservationTerm": "3 Years",
"retailPrice": 6471.0,
"unitPrice": 6471.0,
"armRegionName": "westus",
"location": "US West",
"effectiveStartDate": "2021-11-01T00:00:00Z",
"meterId": "9259894d-0c3c-5b5b-a8be-c1de562f0fb0",
"meterName": "E8d v5",
"productId": "DZH318Z08M9R",
"skuId": "DZH318Z08M9R/0227",
"productName": "Virtual Machines Edv5 Series",
"skuName": "Standard_E8d_v5",
"serviceName": "Virtual Machines",
"serviceId": "DZH313Z7MMC8",
"serviceFamily": "Compute",
"unitOfMeasure": "1 Hour",
"type": "Reservation",
"isPrimaryMeterRegion": true,
"armSkuName": "Standard_E8d_v5"
},
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"reservationTerm": "1 Year",
"retailPrice": 40189.0,
"unitPrice": 40189.0,
"armRegionName": "westus",
"location": "US West",
"effectiveStartDate": "2021-11-01T00:00:00Z",
"meterId": "9d58d7dd-0af8-53ef-ac2b-b1abbbc35236",
"meterName": "E96d v5",
"productId": "DZH318Z08M9R",
"skuId": "DZH318Z08M9R/021J",
"productName": "Virtual Machines Edv5 Series",
"skuName": "Standard_E96d_v5",
"serviceName": "Virtual Machines",
"serviceId": "DZH313Z7MMC8",
"serviceFamily": "Compute",
"unitOfMeasure": "1 Hour",
"type": "Reservation",
"isPrimaryMeterRegion": true,
"armSkuName": "Standard_E96d_v5"
},
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"reservationTerm": "3 Years",
"retailPrice": 77654.0,
"unitPrice": 77654.0,
"armRegionName": "westus",
"location": "US West",
"effectiveStartDate": "2021-11-01T00:00:00Z",
"meterId": "9d58d7dd-0af8-53ef-ac2b-b1abbbc35236",
"meterName": "E96d v5",
"productId": "DZH318Z08M9R",
"skuId": "DZH318Z08M9R/021H",
"productName": "Virtual Machines Edv5 Series",
"skuName": "Standard_E96d_v5",
"serviceName": "Virtual Machines",
"serviceId": "DZH313Z7MMC8",
"serviceFamily": "Compute",
"unitOfMeasure": "1 Hour",
"type": "Reservation",
"isPrimaryMeterRegion": true,
"armSkuName": "Standard_E96d_v5"
},
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"reservationTerm": "1 Year",
"retailPrice": 47892.0,
"unitPrice": 47892.0,
"armRegionName": "westus",
"location": "US West",
"effectiveStartDate": "2021-11-01T00:00:00Z",
"meterId": "9db0122c-778f-5584-abaf-b3c5867b9d29",
"meterName": "E104id v5",
"productId": "DZH318Z08M9R",
"skuId": "DZH318Z08M9R/020T",
"productName": "Virtual Machines Edv5 Series",
"skuName": "Standard_E104id_v5",
"serviceName": "Virtual Machines",
"serviceId": "DZH313Z7MMC8",
"serviceFamily": "Compute",
"unitOfMeasure": "1 Hour",
"type": "Reservation",
"isPrimaryMeterRegion": true,
"armSkuName": "Standard_E104id_v5"
},
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"reservationTerm": "3 Years",
"retailPrice": 92538.0,
"unitPrice": 92538.0,
"armRegionName": "westus",
"location": "US West",
"effectiveStartDate": "2021-11-01T00:00:00Z",
"meterId": "9db0122c-778f-5584-abaf-b3c5867b9d29",
"meterName": "E104id v5",
"productId": "DZH318Z08M9R",
"skuId": "DZH318Z08M9R/020W",
"productName": "Virtual Machines Edv5 Series",
"skuName": "Standard_E104id_v5",
"serviceName": "Virtual Machines",
"serviceId": "DZH313Z7MMC8",
"serviceFamily": "Compute",
"unitOfMeasure": "1 Hour",
"type": "Reservation",
"isPrimaryMeterRegion": true,
"armSkuName": "Standard_E104id_v5"
},
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"reservationTerm": "3 Years",
"retailPrice": 38827.0,
"unitPrice": 38827.0,
"armRegionName": "westus",
"location": "US West",
"effectiveStartDate": "2021-11-01T00:00:00Z",
"meterId": "c4fd25cd-fc1d-5273-942c-1f2814f3546f",
"meterName": "E48d v5",
"productId": "DZH318Z08M9R",
"skuId": "DZH318Z08M9R/021K",
"productName": "Virtual Machines Edv5 Series",
"skuName": "Standard_E48d_v5",
"serviceName": "Virtual Machines",
"serviceId": "DZH313Z7MMC8",
"serviceFamily": "Compute",
"unitOfMeasure": "1 Hour",
"type": "Reservation",
"isPrimaryMeterRegion": true,
"armSkuName": "Standard_E48d_v5"
},
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"reservationTerm": "1 Year",
"retailPrice": 20095.0,
"unitPrice": 20095.0,
"armRegionName": "westus",
"location": "US West",
"effectiveStartDate": "2021-11-01T00:00:00Z",
"meterId": "c4fd25cd-fc1d-5273-942c-1f2814f3546f",
"meterName": "E48d v5",
"productId": "DZH318Z08M9R",
"skuId": "DZH318Z08M9R/021P",
"productName": "Virtual Machines Edv5 Series",
"skuName": "Standard_E48d_v5",
"serviceName": "Virtual Machines",
"serviceId": "DZH313Z7MMC8",
"serviceFamily": "Compute",
"unitOfMeasure": "1 Hour",
"type": "Reservation",
"isPrimaryMeterRegion": true,
"armSkuName": "Standard_E48d_v5"
},
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"reservationTerm": "1 Year",
"retailPrice": 6698.0,
"unitPrice": 6698.0,
"armRegionName": "westus",
"location": "US West",
"effectiveStartDate": "2021-11-01T00:00:00Z",
"meterId": "d00719f8-10b0-591d-ac3e-ca774a3872eb",
"meterName": "E16d v5",
"productId": "DZH318Z08M9R",
"skuId": "DZH318Z08M9R/0225",
"productName": "Virtual Machines Edv5 Series",
"skuName": "Standard_E16d_v5",
"serviceName": "Virtual Machines",
"serviceId": "DZH313Z7MMC8",
"serviceFamily": "Compute",
"unitOfMeasure": "1 Hour",
"type": "Reservation",
"isPrimaryMeterRegion": true,
"armSkuName": "Standard_E16d_v5"
},
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"reservationTerm": "3 Years",
"retailPrice": 12942.0,
"unitPrice": 12942.0,
"armRegionName": "westus",
"location": "US West",
"effectiveStartDate": "2021-11-01T00:00:00Z",
"meterId": "d00719f8-10b0-591d-ac3e-ca774a3872eb",
"meterName": "E16d v5",
"productId": "DZH318Z08M9R",
"skuId": "DZH318Z08M9R/021W",
"productName": "Virtual Machines Edv5 Series",
"skuName": "Standard_E16d_v5",
"serviceName": "Virtual Machines",
"serviceId": "DZH313Z7MMC8",
"serviceFamily": "Compute",
"unitOfMeasure": "1 Hour",
"type": "Reservation",
"isPrimaryMeterRegion": true,
"armSkuName": "Standard_E16d_v5"
},
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"reservationTerm": "3 Years",
"retailPrice": 16178.0,
"unitPrice": 16178.0,
"armRegionName": "westus",
"location": "US West",
"effectiveStartDate": "2021-11-01T00:00:00Z",
"meterId": "d20aa38f-459d-5a1b-8bd3-351220027f18",
"meterName": "E20d v5",
"productId": "DZH318Z08M9R",
"skuId": "DZH318Z08M9R/021R",
"productName": "Virtual Machines Edv5 Series",
"skuName": "Standard_E20d_v5",
"serviceName": "Virtual Machines",
"serviceId": "DZH313Z7MMC8",
"serviceFamily": "Compute",
"unitOfMeasure": "1 Hour",
"type": "Reservation",
"isPrimaryMeterRegion": true,
"armSkuName": "Standard_E20d_v5"
},
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"reservationTerm": "1 Year",
"retailPrice": 8373.0,
"unitPrice": 8373.0,
"armRegionName": "westus",
"location": "US West",
"effectiveStartDate": "2021-11-01T00:00:00Z",
"meterId": "d20aa38f-459d-5a1b-8bd3-351220027f18",
"meterName": "E20d v5",
"productId": "DZH318Z08M9R",
"skuId": "DZH318Z08M9R/0223",
"productName": "Virtual Machines Edv5 Series",
"skuName": "Standard_E20d_v5",
"serviceName": "Virtual Machines",
"serviceId": "DZH313Z7MMC8",
"serviceFamily": "Compute",
"unitOfMeasure": "1 Hour",
"type": "Reservation",
"isPrimaryMeterRegion": true,
"armSkuName": "Standard_E20d_v5"
},
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"reservationTerm": "1 Year",
"retailPrice": 13396.0,
"unitPrice": 13396.0,
"armRegionName": "westus",
"location": "US West",
"effectiveStartDate": "2021-11-01T00:00:00Z",
"meterId": "dd03c572-fc78-5cf2-afc3-b4d7fc3663ef",
"meterName": "E32d v5",
"productId": "DZH318Z08M9R",
"skuId": "DZH318Z08M9R/0218",
"productName": "Virtual Machines Edv5 Series",
"skuName": "Standard_E32d_v5",
"serviceName": "Virtual Machines",
"serviceId": "DZH313Z7MMC8",
"serviceFamily": "Compute",
"unitOfMeasure": "1 Hour",
"type": "Reservation",
"isPrimaryMeterRegion": true,
"armSkuName": "Standard_E32d_v5"
},
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"reservationTerm": "3 Years",
"retailPrice": 25885.0,
"unitPrice": 25885.0,
"armRegionName": "westus",
"location": "US West",
"effectiveStartDate": "2021-11-01T00:00:00Z",
"meterId": "dd03c572-fc78-5cf2-afc3-b4d7fc3663ef",
"meterName": "E32d v5",
"productId": "DZH318Z08M9R",
"skuId": "DZH318Z08M9R/0216",
"productName": "Virtual Machines Edv5 Series",
"skuName": "Standard_E32d_v5",
"serviceName": "Virtual Machines",
"serviceId": "DZH313Z7MMC8",
"serviceFamily": "Compute",
"unitOfMeasure": "1 Hour",
"type": "Reservation",
"isPrimaryMeterRegion": true,
"armSkuName": "Standard_E32d_v5"
},
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"reservationTerm": "3 Years",
"retailPrice": 3236.0,
"unitPrice": 3236.0,
"armRegionName": "westus",
"location": "US West",
"effectiveStartDate": "2021-11-01T00:00:00Z",
"meterId": "e8bfdb6f-4c16-5245-a11d-270ff85537f1",
"meterName": "E4d v5",
"productId": "DZH318Z08M9R",
"skuId": "DZH318Z08M9R/0228",
"productName": "Virtual Machines Edv5 Series",
"skuName": "Standard_E4d_v5",
"serviceName": "Virtual Machines",
"serviceId": "DZH313Z7MMC8",
"serviceFamily": "Compute",
"unitOfMeasure": "1 Hour",
"type": "Reservation",
"isPrimaryMeterRegion": true,
"armSkuName": "Standard_E4d_v5"
},
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"reservationTerm": "1 Year",
"retailPrice": 1675.0,
"unitPrice": 1675.0,
"armRegionName": "westus",
"location": "US West",
"effectiveStartDate": "2021-11-01T00:00:00Z",
"meterId": "e8bfdb6f-4c16-5245-a11d-270ff85537f1",
"meterName": "E4d v5",
"productId": "DZH318Z08M9R",
"skuId": "DZH318Z08M9R/022D",
"productName": "Virtual Machines Edv5 Series",
"skuName": "Standard_E4d_v5",
"serviceName": "Virtual Machines",
"serviceId": "DZH313Z7MMC8",
"serviceFamily": "Compute",
"unitOfMeasure": "1 Hour",
"type": "Reservation",
"isPrimaryMeterRegion": true,
"armSkuName": "Standard_E4d_v5"
},
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"reservationTerm": "1 Year",
"retailPrice": 837.0,
"unitPrice": 837.0,
"armRegionName": "westus",
"location": "US West",
"effectiveStartDate": "2021-11-01T00:00:00Z",
"meterId": "fe571f81-3619-5588-8d68-cf1ed70a5297",
"meterName": "E2d v5",
"productId": "DZH318Z08M9R",
"skuId": "DZH318Z08M9R/021S",
"productName": "Virtual Machines Edv5 Series",
"skuName": "Standard_E2d_v5",
"serviceName": "Virtual Machines",
"serviceId": "DZH313Z7MMC8",
"serviceFamily": "Compute",
"unitOfMeasure": "1 Hour",
"type": "Reservation",
"isPrimaryMeterRegion": true,
"armSkuName": "Standard_E2d_v5"
},
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"reservationTerm": "3 Years",
"retailPrice": 1618.0,
"unitPrice": 1618.0,
"armRegionName": "westus",
"location": "US West",
"effectiveStartDate": "2021-11-01T00:00:00Z",
"meterId": "fe571f81-3619-5588-8d68-cf1ed70a5297",
"meterName": "E2d v5",
"productId": "DZH318Z08M9R",
"skuId": "DZH318Z08M9R/021C",
"productName": "Virtual Machines Edv5 Series",
"skuName": "Standard_E2d_v5",
"serviceName": "Virtual Machines",
"serviceId": "DZH313Z7MMC8",
"serviceFamily": "Compute",
"unitOfMeasure": "1 Hour",
"type": "Reservation",
"isPrimaryMeterRegion": true,
"armSkuName": "Standard_E2d_v5"
}
],
"NextPageLink": null,
"Count": 20
}
Example Database Service:
Example Program #4
This program queries the Azure Retail Price APIs and fetches the price for services associated with Azure Cosmos DB in the US West region. The program saves the output as csv and json files on the computer.
#!/usr/bin/env python3
import requests
import json
from tabulate import tabulate
import pandas as pd
import os
import csv
def build_pricing_table(json_data, table_data):
for item in json_data["Items"]:
table_data.append(
[
item["armRegionName"],
item["location"],
item["meterName"],
item["serviceName"],
item["productName"],
item["skuName"],
item["currencyCode"],
item["retailPrice"],
item["unitOfMeasure"],
item["type"],
]
)
def main():
table_data = []
table_data.append(
[
"Region Name",
"Region",
"Meter",
"Service Name",
"Product Name",
"SKU Name",
"Currency Code",
"Retail Price",
"Unit of Measure",
"Price Type",
]
)
api_url = (
"https://prices.azure.com/api/retail/prices?api-version=2023-01-01-preview"
)
query = "armRegionName eq 'westus' and serviceFamily eq 'Databases' and serviceName eq 'Azure Cosmos DB' and productName eq 'Azure Cosmos DB'"
response = requests.get(api_url, params={"$filter": query})
json_data = json.loads(response.text)
build_pricing_table(json_data, table_data)
nextPage = json_data["NextPageLink"]
while nextPage:
response = requests.get(nextPage)
json_data = json.loads(response.text)
nextPage = json_data["NextPageLink"]
build_pricing_table(json_data, table_data)
# Print pricing data
print(tabulate(table_data, headers="firstrow", tablefmt="psql"))
# Set your file name to save the json to a csv file
csvfilename = "AzureDBPrice.csv"
jsonfilename = "AzureDBPrice.json"
# Save the price list data in json format to a data frame
with open(jsonfilename, "w", encoding="utf-8") as f:
json.dump(json_data, f, ensure_ascii=False, indent=4)
# save the price list data in a csv file
price_df = pd.DataFrame(table_data)
price_df.to_csv(csvfilename, index=False, header=False)
if __name__ == "__main__":
main()
Output:
CSV file:

JSON file:
{
"BillingCurrency": "USD",
"CustomerEntityId": "Default",
"CustomerEntityType": "Retail",
"Items": [
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"retailPrice": 0.016,
"unitPrice": 0.016,
"armRegionName": "westus",
"location": "US West",
"effectiveStartDate": "2018-09-24T00:00:00Z",
"meterId": "09b7e759-a6ba-4995-bf46-266678bf06cc",
"meterName": "100 Multi-master RU/s",
"productId": "DZH318Z0BPJH",
"skuId": "DZH318Z0BPJH/00CR",
"productName": "Azure Cosmos DB",
"skuName": "mRUs",
"serviceName": "Azure Cosmos DB",
"serviceId": "DZH317DL9CC7",
"serviceFamily": "Databases",
"unitOfMeasure": "1/Hour",
"type": "Consumption",
"isPrimaryMeterRegion": true,
"armSkuName": ""
},
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"retailPrice": 0.008,
"unitPrice": 0.008,
"armRegionName": "westus",
"location": "US West",
"effectiveStartDate": "2016-04-01T00:00:00Z",
"meterId": "38529ded-5b8e-4b49-b078-ce81794a3543",
"meterName": "100 RU/s",
"productId": "DZH318Z0BPJH",
"skuId": "DZH318Z0BPJH/001K",
"productName": "Azure Cosmos DB",
"skuName": "RUs",
"serviceName": "Azure Cosmos DB",
"serviceId": "DZH317DL9CC7",
"serviceFamily": "Databases",
"unitOfMeasure": "1/Hour",
"type": "Consumption",
"isPrimaryMeterRegion": true,
"armSkuName": ""
},
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"retailPrice": 0.0,
"unitPrice": 0.0,
"armRegionName": "westus",
"location": "US West",
"effectiveStartDate": "2021-07-01T00:00:00Z",
"meterId": "5b0cfcf0-8876-5303-89d5-a0473c6e135f",
"meterName": "100 Multi-master RU/s",
"productId": "DZH318Z0BPJH",
"skuId": "DZH318Z0BPJH/0108",
"productName": "Azure Cosmos DB",
"skuName": "Free Tier",
"serviceName": "Azure Cosmos DB",
"serviceId": "DZH317DL9CC7",
"serviceFamily": "Databases",
"unitOfMeasure": "1/Hour",
"type": "Consumption",
"isPrimaryMeterRegion": true,
"armSkuName": ""
},
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"retailPrice": 0.0,
"unitPrice": 0.0,
"armRegionName": "westus",
"location": "US West",
"effectiveStartDate": "2021-07-01T00:00:00Z",
"meterId": "5bcb1eef-e351-5428-9173-94fef9244dba",
"meterName": "100 RU/s",
"productId": "DZH318Z0BPJH",
"skuId": "DZH318Z0BPJH/0108",
"productName": "Azure Cosmos DB",
"skuName": "Free Tier",
"serviceName": "Azure Cosmos DB",
"serviceId": "DZH317DL9CC7",
"serviceFamily": "Databases",
"unitOfMeasure": "1/Hour",
"type": "Consumption",
"isPrimaryMeterRegion": true,
"armSkuName": ""
},
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"retailPrice": 0.0,
"unitPrice": 0.0,
"armRegionName": "westus",
"location": "US West",
"effectiveStartDate": "2021-07-01T00:00:00Z",
"meterId": "8368dfbe-9661-5eb0-b347-050fc9835411",
"meterName": "Data Stored",
"productId": "DZH318Z0BPJH",
"skuId": "DZH318Z0BPJH/0108",
"productName": "Azure Cosmos DB",
"skuName": "Free Tier",
"serviceName": "Azure Cosmos DB",
"serviceId": "DZH317DL9CC7",
"serviceFamily": "Databases",
"unitOfMeasure": "1 GB/Month",
"type": "Consumption",
"isPrimaryMeterRegion": true,
"armSkuName": ""
},
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"retailPrice": 0.0,
"unitPrice": 0.0,
"armRegionName": "westus",
"location": "US West",
"effectiveStartDate": "2018-09-24T00:00:00Z",
"meterId": "e7a3800b-837b-46df-aa29-147ccf373cfb",
"meterName": "Free 100 Multi-master RU/s",
"productId": "DZH318Z0BPJH",
"skuId": "DZH318Z0BPJH/001J",
"productName": "Azure Cosmos DB",
"skuName": "Free",
"serviceName": "Azure Cosmos DB",
"serviceId": "DZH317DL9CC7",
"serviceFamily": "Databases",
"unitOfMeasure": "1/Hour",
"type": "Consumption",
"isPrimaryMeterRegion": false,
"armSkuName": ""
},
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"retailPrice": 0.25,
"unitPrice": 0.25,
"armRegionName": "westus",
"location": "US West",
"effectiveStartDate": "2016-04-01T00:00:00Z",
"meterId": "ec40c4c2-c5db-4c7c-991f-41d31d8f2bf9",
"meterName": "Data Stored",
"productId": "DZH318Z0BPJH",
"skuId": "DZH318Z0BPJH/00CR",
"productName": "Azure Cosmos DB",
"skuName": "mRUs",
"serviceName": "Azure Cosmos DB",
"serviceId": "DZH317DL9CC7",
"serviceFamily": "Databases",
"unitOfMeasure": "1 GB/Month",
"type": "Consumption",
"isPrimaryMeterRegion": false,
"armSkuName": ""
},
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"retailPrice": 0.25,
"unitPrice": 0.25,
"armRegionName": "westus",
"location": "US West",
"effectiveStartDate": "2016-04-01T00:00:00Z",
"meterId": "ec40c4c2-c5db-4c7c-991f-41d31d8f2bf9",
"meterName": "Data Stored",
"productId": "DZH318Z0BPJH",
"skuId": "DZH318Z0BPJH/00GX",
"productName": "Azure Cosmos DB",
"skuName": "RUm",
"serviceName": "Azure Cosmos DB",
"serviceId": "DZH317DL9CC7",
"serviceFamily": "Databases",
"unitOfMeasure": "1 GB/Month",
"type": "Consumption",
"isPrimaryMeterRegion": false,
"armSkuName": ""
},
{
"currencyCode": "USD",
"tierMinimumUnits": 0.0,
"retailPrice": 0.25,
"unitPrice": 0.25,
"armRegionName": "westus",
"location": "US West",
"effectiveStartDate": "2016-04-01T00:00:00Z",
"meterId": "ec40c4c2-c5db-4c7c-991f-41d31d8f2bf9",
"meterName": "Data Stored",
"productId": "DZH318Z0BPJH",
"skuId": "DZH318Z0BPJH/001K",
"productName": "Azure Cosmos DB",
"skuName": "RUs",
"serviceName": "Azure Cosmos DB",
"serviceId": "DZH317DL9CC7",
"serviceFamily": "Databases",
"unitOfMeasure": "1 GB/Month",
"type": "Consumption",
"isPrimaryMeterRegion": true,
"armSkuName": ""
}
],
"NextPageLink": null,
"Count": 9
}
Closing Thoughts:
In this blog, I shared the basics of Azure Retail Prices API. This API can be used in a lot more interesting ways (for example use response data to calculate overall cost of your whole stack), but I covered some of the most important foundational use, especially because once you have data in csv on computer you can do a lot of interesting things in excel as well. Below is how this API can help you:
Selecting the Optimal Cloud Region: Let’s say your product or SaaS service is growing and you are thinking about hosting the service closer to your customers in different geographies such as Europe, or Asia, or Americas etc. apart form availability of services, features, latency, regulatory compliance, and data privacy you need to also consider cost. As cloud services are priced differently from one Region to another, this API provides a programmatic way to build a cost model to compare different regions.
Estimated Cost Forecasting: Let’s say you are launching a new product and building a business case for it. Executive leaders in your organization will likely want to know ROI of the project, health of cash flow, and EBITDA margin. Building out the cost model using online pricing calculators can be complicated and tedious. Therefore these APIs can help you programmatically fetch the relevant information quickly and accurately and remove some of the grunt work associated with building the cost model.
Retrieving Retail Pricing: Last but not the least fetching retail price is foundational use of these APIs. Whether you want to identify low-cost resource opportunities, or do estimation or build a model or do some other even more interesting thing is up to you.
I hope you found this blog useful. Amazon Web Services (AWS) and Google Cloud Platform (GCP) APIs are a little different but you can find the details in AWS API documentation and GCP API documentation respectively.

